C# Mini版本文件浏览器.TreeView/ListView应用
C# Mini版本文件浏览器.TreeView/ListView应用C# Mini版文件浏览器.介绍TreeView及ListView应用
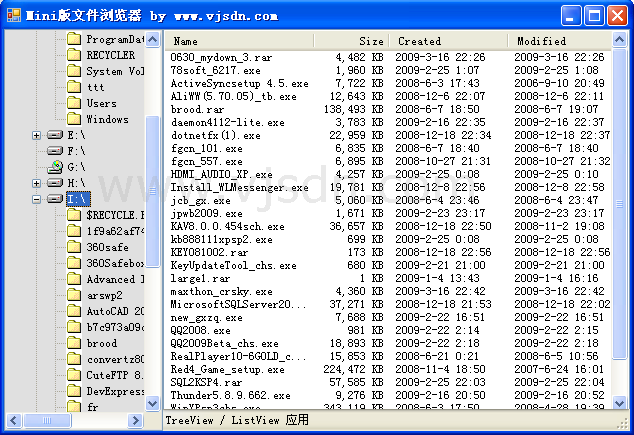
程序内用到System.Management.ManagementObjectCollection
ManagementObjectCollection类说明:
// Summary:
//
// Represents different collections of management objects retrieved through
// WMI. The objects in this collection are of System.Management.ManagementBaseObject-derived
// types, including System.Management.ManagementObject and System.Management.ManagementClass.
// The collection can be the result of a WMI query executed through a System.Management.
// ManagementObjectSearcher,
// or an enumeration of management objects of a specified type retrieved through
// a System.Management.ManagementClass representing that type. In addition,
// this can be a collection of management objects related in a specified way
// to a specific management object - in this case the collection would be retrieved
// through a method such as System.Management.ManagementObject.GetRelated().
// The collection can be walked using the System.Management.ManagementObjectCollection.
// ManagementObjectEnumerator
// and objects in it can be inspected or manipulated for various management
// tasks.
顺便学学英文,总有好处。
下面是窗体源代码,多看看,别下载了源程序运行通过就不管事了。学程序要多看多写多总结---学三多
Source Code:
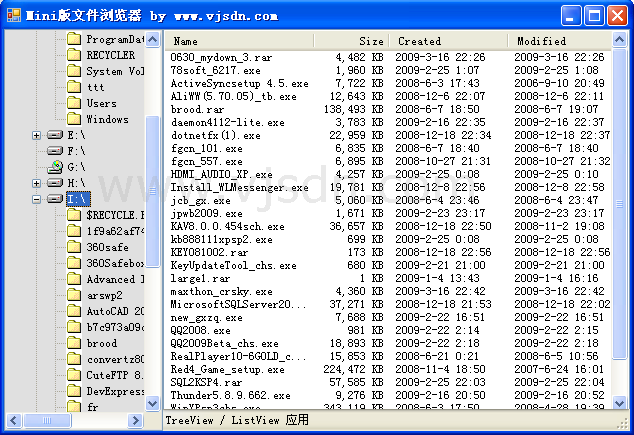
程序内用到System.Management.ManagementObjectCollection
ManagementObjectCollection类说明:
// Summary:
//
// Represents different collections of management objects retrieved through
// WMI. The objects in this collection are of System.Management.ManagementBaseObject-derived
// types, including System.Management.ManagementObject and System.Management.ManagementClass.
// The collection can be the result of a WMI query executed through a System.Management.
// ManagementObjectSearcher,
// or an enumeration of management objects of a specified type retrieved through
// a System.Management.ManagementClass representing that type. In addition,
// this can be a collection of management objects related in a specified way
// to a specific management object - in this case the collection would be retrieved
// through a method such as System.Management.ManagementObject.GetRelated().
// The collection can be walked using the System.Management.ManagementObjectCollection.
// ManagementObjectEnumerator
// and objects in it can be inspected or manipulated for various management
// tasks.
顺便学学英文,总有好处。
下面是窗体源代码,多看看,别下载了源程序运行通过就不管事了。学程序要多看多写多总结---学三多
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Management;
using System.Globalization;
using System.IO;
namespace MiniExplorer
{
//用TreeView实现文件浏览器
public partial class frmMiniExplorer : Form
{
private const int Removable = 2;
private const int LocalDisk = 3;
private const int Network = 4;
private const int CD = 5;
public frmMiniExplorer()
{
InitializeComponent();
}
private void frmMiniExplorer_Load(object sender, EventArgs e)
{
LoadDrives();
LoadListView();
}
//加载物理磁盘驱动器
private void LoadDrives()
{
TreeNode nodeTemp = null;
int imageIndex = 0;
int selectIndex = 0;
this.Cursor = Cursors.WaitCursor;
tvFolders.Nodes.Clear();
nodeTemp = new TreeNode("My Computer", 0, 0);
tvFolders.Nodes.Add(nodeTemp);//加载根目录
TreeNodeCollection nodeCollection = nodeTemp.Nodes;
//用System.Management对象获取驱动器列表
ManagementObjectCollection queryCollection = GetDriveList();
foreach (ManagementObject mo in queryCollection)
{
switch (int.Parse(mo["DriveType"].ToString()))
{
case Removable: //Removable Drives
imageIndex = 5;
selectIndex = 5;
break;
case LocalDisk: //硬盘
imageIndex = 6;
selectIndex = 6;
break;
case CD: //光驱
imageIndex = 7;
selectIndex = 7;
break;
case Network: //网络磁盘
imageIndex = 8;
selectIndex = 8;
break;
default: //预设显示目录图标
imageIndex = 2;
selectIndex = 3;
break;
}
//建立驱动器
nodeTemp = new TreeNode(mo["Name"].ToString() + "\\", imageIndex, selectIndex);
nodeCollection.Add(nodeTemp);
}
this.Cursor = Cursors.Default;
}
private void tvFolders_AfterSelect(object sender, System.Windows.Forms.TreeViewEventArgs e)
{
this.Cursor = Cursors.WaitCursor;
TreeNode nodeCurrent = e.Node;
nodeCurrent.Nodes.Clear();
if (nodeCurrent.SelectedImageIndex == 0)
LoadDrives();
else
LoadDirectory(nodeCurrent, nodeCurrent.Nodes);
this.Cursor = Cursors.Default;
}
protected void LoadListView()
{
lvFiles.Clear();
//创建ListView的标题列
lvFiles.Columns.Add("Name", 150, System.Windows.Forms.HorizontalAlignment.Left);
lvFiles.Columns.Add("Size", 75, System.Windows.Forms.HorizontalAlignment.Right);
lvFiles.Columns.Add("Created", 140, System.Windows.Forms.HorizontalAlignment.Left);
lvFiles.Columns.Add("Modified", 140, System.Windows.Forms.HorizontalAlignment.Left);
}
protected void LoadDirectory(TreeNode nodeCurrent, TreeNodeCollection nodeCurrentCollection)
{
TreeNode nodeTemp = null;
int imageIndex = 2; //当前结点的图片
int selectIndex = 3; //选中结点时显示的图片
if (nodeCurrent.SelectedImageIndex != 0)
{
try
{
if (Directory.Exists(FormatPath(nodeCurrent.FullPath)) == false)
throw new Exception("目录或路路径不存在!");
LoadFiles(nodeCurrent);
string[] stringDirectories = Directory.GetDirectories(FormatPath(nodeCurrent.FullPath));
string stringFullPath = "";
string stringPathName = "";
//加载当前目录下所有子目录
foreach (string stringDir in stringDirectories)
{
stringFullPath = stringDir;
stringPathName = GetPathName(stringFullPath);
TreeNode nodeDir = new TreeNode(stringPathName.ToString(), imageIndex, selectIndex);
nodeCurrentCollection.Add(nodeDir);
}
}
catch (Exception e)
{
MessageBox.Show(e.Message);
}
}
}
protected string GetPathName(string stringPath)
{
string[] stringSplit = stringPath.Split(’\\’);
return stringSplit[stringSplit.Length - 1];
}
//加载当前目录下的文件
protected void LoadFiles(TreeNode nodeCurrent)
{
string[] listviewData = new string[4];
this.LoadListView();
if (nodeCurrent.SelectedImageIndex != 0)
{
if (Directory.Exists((string)FormatPath(nodeCurrent.FullPath)) == false)
throw new Exception("目录或路路径不存在!");
try
{
string[] stringFiles = Directory.GetFiles(FormatPath(nodeCurrent.FullPath));
DateTime dtCreateDate, dtModifyDate;
Int64 lFileSize = 0;
foreach (string stringFile in stringFiles)
{
FileInfo objFileSize = new FileInfo(stringFile);
lFileSize = objFileSize.Length;
dtCreateDate = objFileSize.CreationTime;
dtModifyDate = objFileSize.LastWriteTime;
listviewData[0] = GetPathName(stringFile);
listviewData[1] = FormatSize(lFileSize);
if (TimeZone.CurrentTimeZone.IsDaylightSavingTime(dtCreateDate) == false)
listviewData[2] = FormatDate(dtCreateDate.AddHours(1));
else
listviewData[2] = FormatDate(dtCreateDate);
if (TimeZone.CurrentTimeZone.IsDaylightSavingTime(dtModifyDate) == false)
listviewData[3] = FormatDate(dtModifyDate.AddHours(1));
else
listviewData[3] = FormatDate(dtModifyDate);
ListViewItem lvItem = new ListViewItem(listviewData, 0);
lvFiles.Items.Add(lvItem);
}
}
catch (Exception e)
{
MessageBox.Show(e.Message);
}
}
}
//替代Root是My Computer
protected string FormatPath(string stringPath)
{
string stringParse = stringPath.Replace("My Computer\\", "");
return stringParse;
}
//获取所有磁盘驱器
protected ManagementObjectCollection GetDriveList()
{
ManagementObjectSearcher query = new ManagementObjectSearcher("SELECT * From Win32_LogicalDisk ");
ManagementObjectCollection queryCollection = query.Get();
return queryCollection;
}
//转换为短日期格式
protected string FormatDate(DateTime dtDate)
{
string stringDate = "";
stringDate = dtDate.ToShortDateString().ToString() + " " + dtDate.ToShortTimeString().ToString();
return stringDate;
}
//将文件大小转换为带KB的字符串
protected string FormatSize(Int64 lSize)
{
string stringSize = "";
NumberFormatInfo numInfo = new NumberFormatInfo();
if (lSize < 1024) //小于1KB
{
if (lSize == 0)
stringSize = "0";
else
stringSize = "1";
}
else
{
Int64 lKBSize = lSize / 1024; //转折为MB / 1024
stringSize = lKBSize.ToString("n", numInfo);
stringSize = stringSize.Replace(".00", "");
}
return stringSize + " KB";
}
}
}
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Management;
using System.Globalization;
using System.IO;
namespace MiniExplorer
{
//用TreeView实现文件浏览器
public partial class frmMiniExplorer : Form
{
private const int Removable = 2;
private const int LocalDisk = 3;
private const int Network = 4;
private const int CD = 5;
public frmMiniExplorer()
{
InitializeComponent();
}
private void frmMiniExplorer_Load(object sender, EventArgs e)
{
LoadDrives();
LoadListView();
}
//加载物理磁盘驱动器
private void LoadDrives()
{
TreeNode nodeTemp = null;
int imageIndex = 0;
int selectIndex = 0;
this.Cursor = Cursors.WaitCursor;
tvFolders.Nodes.Clear();
nodeTemp = new TreeNode("My Computer", 0, 0);
tvFolders.Nodes.Add(nodeTemp);//加载根目录
TreeNodeCollection nodeCollection = nodeTemp.Nodes;
//用System.Management对象获取驱动器列表
ManagementObjectCollection queryCollection = GetDriveList();
foreach (ManagementObject mo in queryCollection)
{
switch (int.Parse(mo["DriveType"].ToString()))
{
case Removable: //Removable Drives
imageIndex = 5;
selectIndex = 5;
break;
case LocalDisk: //硬盘
imageIndex = 6;
selectIndex = 6;
break;
case CD: //光驱
imageIndex = 7;
selectIndex = 7;
break;
case Network: //网络磁盘
imageIndex = 8;
selectIndex = 8;
break;
default: //预设显示目录图标
imageIndex = 2;
selectIndex = 3;
break;
}
//建立驱动器
nodeTemp = new TreeNode(mo["Name"].ToString() + "\\", imageIndex, selectIndex);
nodeCollection.Add(nodeTemp);
}
this.Cursor = Cursors.Default;
}
private void tvFolders_AfterSelect(object sender, System.Windows.Forms.TreeViewEventArgs e)
{
this.Cursor = Cursors.WaitCursor;
TreeNode nodeCurrent = e.Node;
nodeCurrent.Nodes.Clear();
if (nodeCurrent.SelectedImageIndex == 0)
LoadDrives();
else
LoadDirectory(nodeCurrent, nodeCurrent.Nodes);
this.Cursor = Cursors.Default;
}
protected void LoadListView()
{
lvFiles.Clear();
//创建ListView的标题列
lvFiles.Columns.Add("Name", 150, System.Windows.Forms.HorizontalAlignment.Left);
lvFiles.Columns.Add("Size", 75, System.Windows.Forms.HorizontalAlignment.Right);
lvFiles.Columns.Add("Created", 140, System.Windows.Forms.HorizontalAlignment.Left);
lvFiles.Columns.Add("Modified", 140, System.Windows.Forms.HorizontalAlignment.Left);
}
protected void LoadDirectory(TreeNode nodeCurrent, TreeNodeCollection nodeCurrentCollection)
{
TreeNode nodeTemp = null;
int imageIndex = 2; //当前结点的图片
int selectIndex = 3; //选中结点时显示的图片
if (nodeCurrent.SelectedImageIndex != 0)
{
try
{
if (Directory.Exists(FormatPath(nodeCurrent.FullPath)) == false)
throw new Exception("目录或路路径不存在!");
LoadFiles(nodeCurrent);
string[] stringDirectories = Directory.GetDirectories(FormatPath(nodeCurrent.FullPath));
string stringFullPath = "";
string stringPathName = "";
//加载当前目录下所有子目录
foreach (string stringDir in stringDirectories)
{
stringFullPath = stringDir;
stringPathName = GetPathName(stringFullPath);
TreeNode nodeDir = new TreeNode(stringPathName.ToString(), imageIndex, selectIndex);
nodeCurrentCollection.Add(nodeDir);
}
}
catch (Exception e)
{
MessageBox.Show(e.Message);
}
}
}
protected string GetPathName(string stringPath)
{
string[] stringSplit = stringPath.Split(’\\’);
return stringSplit[stringSplit.Length - 1];
}
//加载当前目录下的文件
protected void LoadFiles(TreeNode nodeCurrent)
{
string[] listviewData = new string[4];
this.LoadListView();
if (nodeCurrent.SelectedImageIndex != 0)
{
if (Directory.Exists((string)FormatPath(nodeCurrent.FullPath)) == false)
throw new Exception("目录或路路径不存在!");
try
{
string[] stringFiles = Directory.GetFiles(FormatPath(nodeCurrent.FullPath));
DateTime dtCreateDate, dtModifyDate;
Int64 lFileSize = 0;
foreach (string stringFile in stringFiles)
{
FileInfo objFileSize = new FileInfo(stringFile);
lFileSize = objFileSize.Length;
dtCreateDate = objFileSize.CreationTime;
dtModifyDate = objFileSize.LastWriteTime;
listviewData[0] = GetPathName(stringFile);
listviewData[1] = FormatSize(lFileSize);
if (TimeZone.CurrentTimeZone.IsDaylightSavingTime(dtCreateDate) == false)
listviewData[2] = FormatDate(dtCreateDate.AddHours(1));
else
listviewData[2] = FormatDate(dtCreateDate);
if (TimeZone.CurrentTimeZone.IsDaylightSavingTime(dtModifyDate) == false)
listviewData[3] = FormatDate(dtModifyDate.AddHours(1));
else
listviewData[3] = FormatDate(dtModifyDate);
ListViewItem lvItem = new ListViewItem(listviewData, 0);
lvFiles.Items.Add(lvItem);
}
}
catch (Exception e)
{
MessageBox.Show(e.Message);
}
}
}
//替代Root是My Computer
protected string FormatPath(string stringPath)
{
string stringParse = stringPath.Replace("My Computer\\", "");
return stringParse;
}
//获取所有磁盘驱器
protected ManagementObjectCollection GetDriveList()
{
ManagementObjectSearcher query = new ManagementObjectSearcher("SELECT * From Win32_LogicalDisk ");
ManagementObjectCollection queryCollection = query.Get();
return queryCollection;
}
//转换为短日期格式
protected string FormatDate(DateTime dtDate)
{
string stringDate = "";
stringDate = dtDate.ToShortDateString().ToString() + " " + dtDate.ToShortTimeString().ToString();
return stringDate;
}
//将文件大小转换为带KB的字符串
protected string FormatSize(Int64 lSize)
{
string stringSize = "";
NumberFormatInfo numInfo = new NumberFormatInfo();
if (lSize < 1024) //小于1KB
{
if (lSize == 0)
stringSize = "0";
else
stringSize = "1";
}
else
{
Int64 lKBSize = lSize / 1024; //转折为MB / 1024
stringSize = lKBSize.ToString("n", numInfo);
stringSize = stringSize.Replace(".00", "");
}
return stringSize + " KB";
}
}
}
Source Code:
版权声明:本文为开发框架文库发布内容,转载请附上原文出处连接
NewDoc C/S框架网